Overview
This portfolio documents my contributions towards the project, IchiFund, which is part of a software engineering module, CS2103T. My team decided to morph an existing address book software into a desktop finance management application for university students who prefer using a command-line interface. We call this application IchiFund.
My role was to develop the Budgets component and design the user interface for the application.
Summary of contributions
-
Major enhancement: Developed the
Model
,Logic
,Storage
, andUi
infrastructure for budgeting features.-
Functionality: Provides the foundation on which features related to budget, such as
add
,delete
, andfind
, are built upon. -
Justification: The ability to read, create, delete, and find budgets on the application depends on the budget infrastructure. With this infrastructure in place, it is easy to implement and extend the budgeting features within IchiFund.
-
Highlights: This enhancement is part of the core features in IchiFund. The ability to implement this enhancement demonstrates a deep understanding of the underlying MVC design pattern of the original addressbook3 codebase. This is clearly highlighted when I introduced a
Task
abstraction to update all budgets upon an update of any transaction.
-
-
Minor enhancement:
-
Code contributed: Click here to view the code that I have contributed.
-
Other contributions:
-
Project management:
-
Managed pull requests (PRs) and issue tracker.
-
Refactored existing code of Address Book 3 to IchiFund (PR #83).
-
Protected the master branch.
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Tools:
-
Integrated Travis to the team repository.
-
Integrated a new Github plugin (SlackBot) to the team repository.
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Creating monthly budget: add
Format: add de/DESCRIPTION a/AMOUNT [c/CATEGORY] [m/MONTH y/YEAR]
You can use the add
command to create a budget.
Let us first walk through the process of creating a budget for tracking your spending on food.
-
Check to make sure you are in the budgets tab. If not, switch to it.
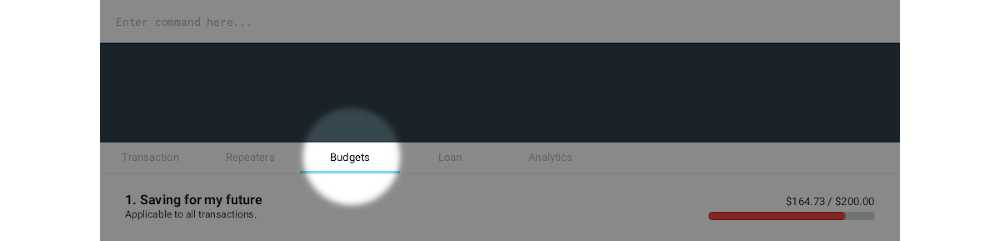
-
Type
add de/Saving my tummy a/82.69 c/food
into the command bar. This will create an $82.69 budget named Saving my tummy that tracks this month’s expenditures tagged with the food category.
If the optional arguments m/MONTH and y/YEAR are not provided, the budget will track all transactions.
|
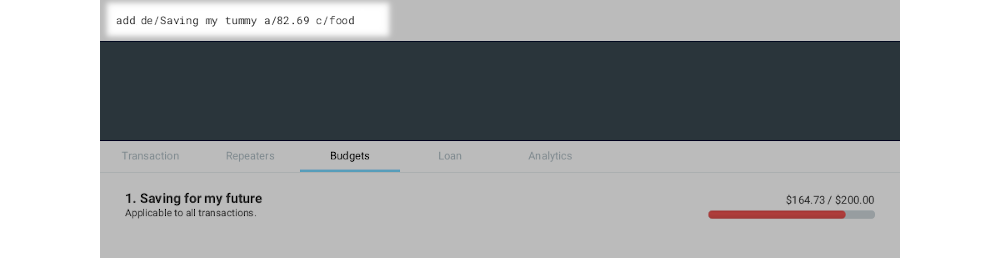
-
Press enter to run the command. You should see the newly created budget on the budget list.
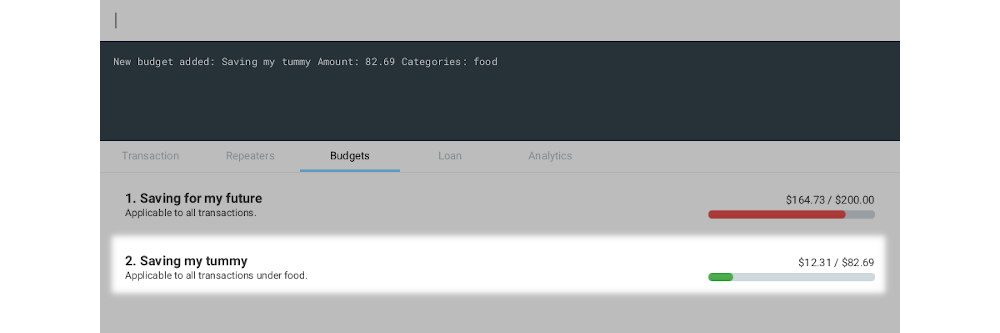
If you want to track all monthly expenditures, simply leave out the c/CATEGORY
argument.
For instance, the following command creates a $500.00 budget that applies to all expenditures:
add de/General budgeting a/500
.
Deleting monthly budget: delete
Format: delete INDEX
You can use delete
to delete a budget with a given index.
Let us walk through the process of deleting the Saving my tummy budget we created previously.
-
Identify the index of the budget you are deleting. Here, the index is 2.
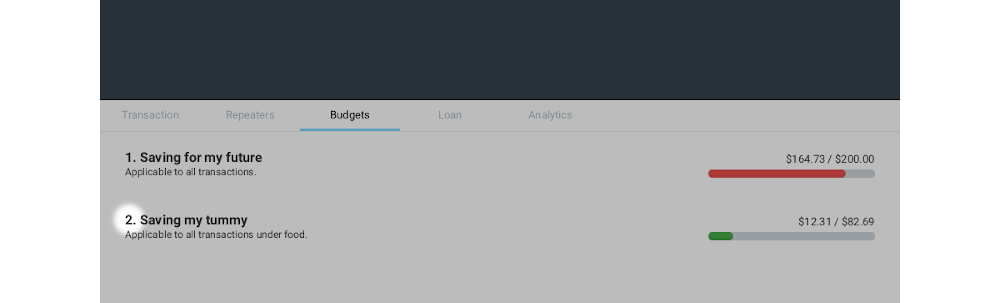
-
Type
delete 2
into the command bar and press enter. You should see the Saving my tummy budget disappear from the list.
Finding monthly budget by keywords: find
Format: find [KEYWORDS]…
You can use find
to find budgets with description that matches all the given keywords.
To illustrate this, let us suppose you want to find all budgets with saving in its description.
To do so, type find saving
into the command bar and press enter.
You should see the search result on the budget list.
If you are unsure about which keywords the budget you are looking for contains, you can enter multiple keywords separated by space. IchiFund will return to you all results that match with at least one keyword. |
You can also revert the budget list to show all budgets by executing find
without any argument.
To revert the budget list so that all budgets are listed, simply execute find
without any argument.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Logic Component
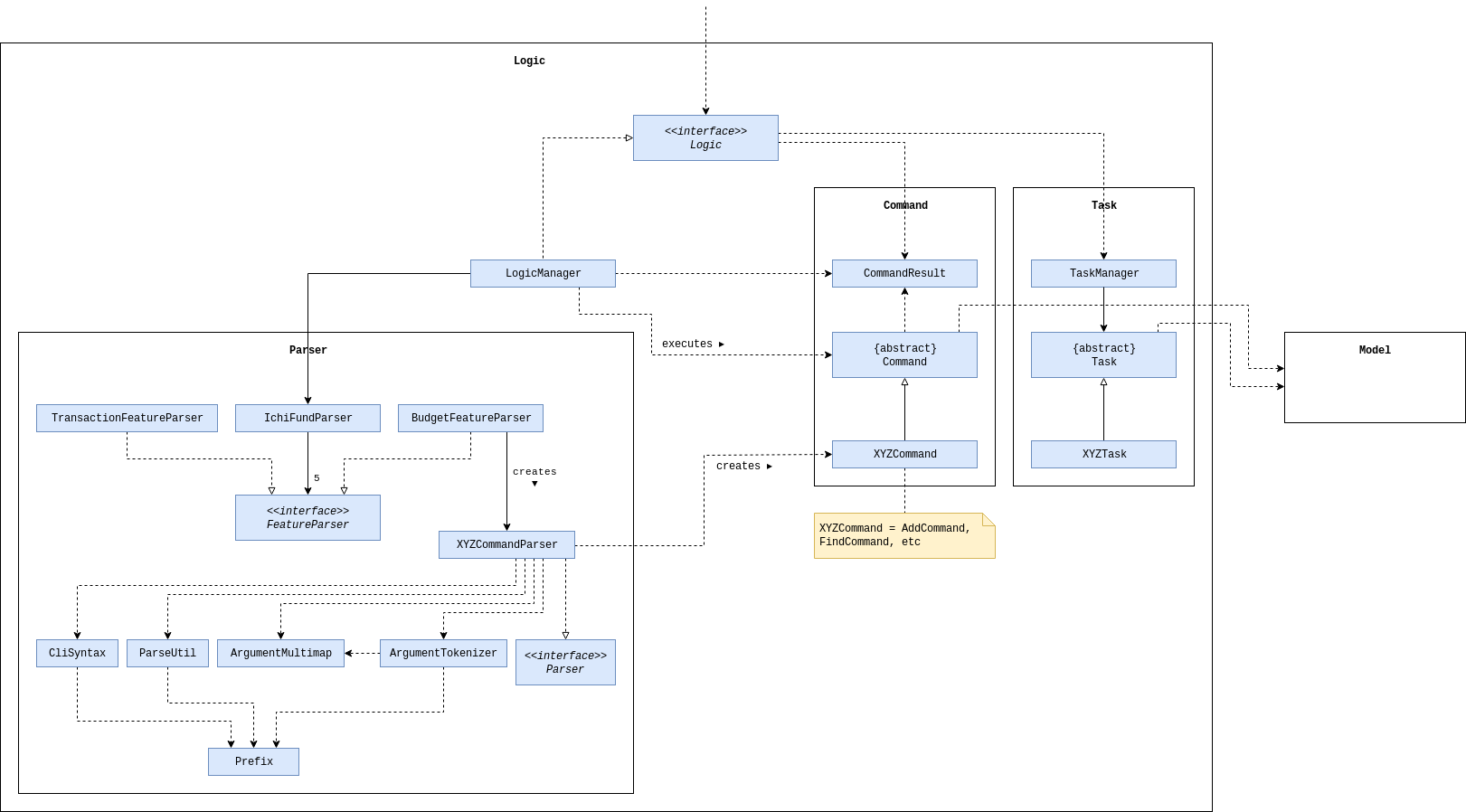
API :
Logic.java
-
Logic
uses theIchiFundParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a person). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
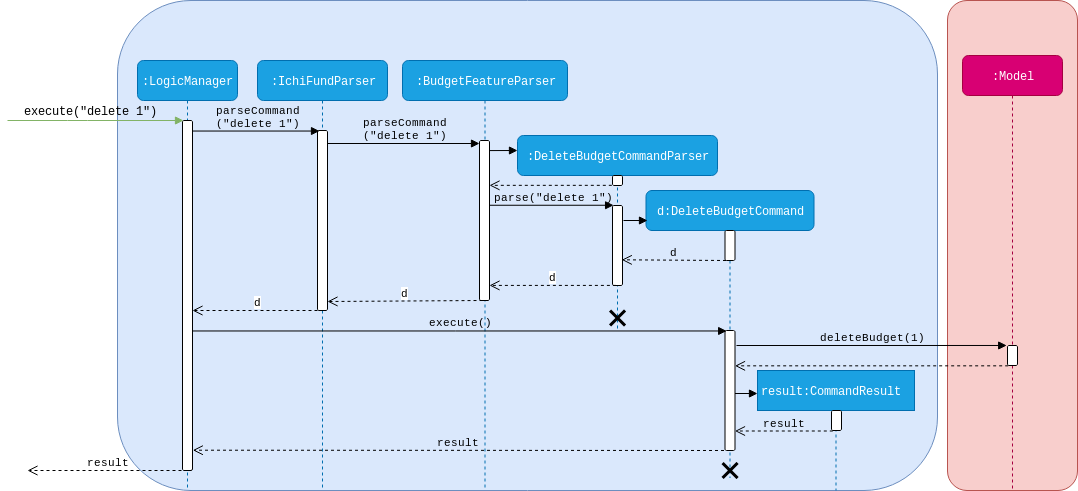
delete 1
CommandTasks
Some models in IchiFund must be refreshed after a command is executed.
For instance, when a new Transaction
is added, all Budget
must be recomputed.
Task
can be used to facilitate such updates.
Implementation
This feature is managed by TaskManager
.
The role of TaskManager
is to maintain a list of all active Task
.
The LogicManager
holds an instance of the TaskManager
.
When the LogicManager#execute()
is called, the following chain of operations occurs:
-
After
Command#execute()
is completed,TaskManager#executeAll()
is called.

-
TaskManager#executeAll()
will iterate through all activeTask
and call the respectiveTask#execute()
method.
Adding Budget
The add budget feature allows the user to add a budget into IchiFund.
This feature is facilitated by BudgetFeatureParser
, AddBudgetCommandParser
, and AddBudgetCommand
.
The arguments supported by this feature includes:
-
Description
-
Amount
-
Category
(optional) -
Month
(optional) -
Year
(optional)
Implementation
When the user input the add
command in the Budget tab, the following chain of operations occurs:
-
The
IchiFundParser
will delegate the parsing of the command toBudgetFeatureParser
if the current active tab is Budget. -
The
BudgetFeatureParser
will delegate the parsing of the arguments toAddBudgetCommandParser
. -
AddBudgetCommandParser#parse()
will take in aString
input consisting of the arguments. -
This arguments will be tokenized and the respective models for each argument are created.
-
If the parsing of all arguments are successful, a new
Budget
object is created using the arguments, and a newAddBudgetCommand
is returned back toLogicManager
. -
The
LogicManager
executesAddBudgetCommand#execute()
. -
The newly created
Budget
is added to the model.
This process is further illustrated in the following sequence diagram:
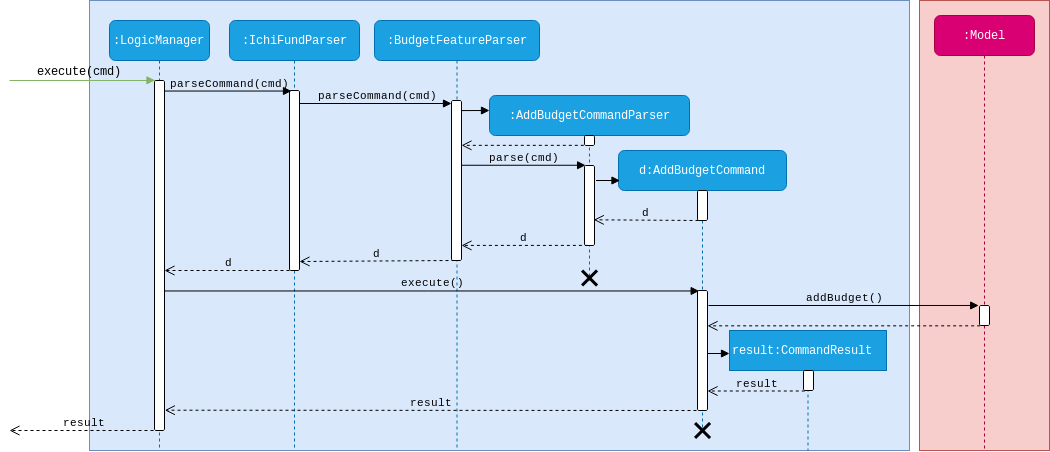
add
Command under Budget Tab